#
Push classification tree
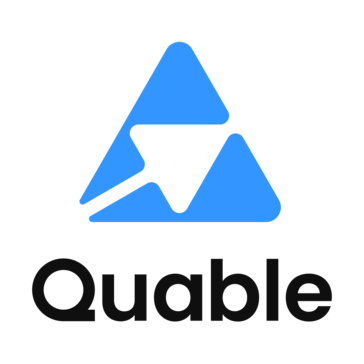
Pushing classifications works the same way for documents and for assets. You can refer to the process below to import both. The import of classifications is optional for assets.
In order to import the hierarchy from your ERP, you will need to create and manage each of the individual classification hierarchy levels. To create the hierarchy shown below, you need to create the top levels first and the lower (sub)levels second.
#
Classifications synchronisation
In accordance with the implementation you define, your hierarchy in Quable PIM will possibily be identical to your ERP categories.
Once this is set, you should consider synchronizing your classification hierarchy in order to place your documents in it.
There are two typical scenarios:
#
Available endpoints overview
Let's say your ERP has documents in the breadcrumb "Tops > Sweaters". You can choose to create 2 classifications :
- classification_tops
- classification_sweaters
Below are the available endpoints to add, get or edit classifications:
Gather all the classifications for the PIM catalog:
GET
/api/classifications?catalogs.id[]=PIM
Get a specific classification, if it already exists:
GET
/api/classifications/classification_sweaters
Create a new classification, if it does not exist already:
POST
/api/classifications
Update an existing classification:
PUT
/api/classifications/classification_sweaters
Delete an existing classification:
DELETE
/api/classifications/classification_sweaters
#
Create classifications
To create classifications, you must know :
- where it will be located in the hierarchy,
- its unique identifier
- its parent classification's unique identifier
You can also include attributes' values for the classification, in every locale.
#
Endpoint
POST /api/classifications
This endpoint is unitary
This endpoint is unitary and creates a single resource at a time.
#
Body parameters
#
Example
import requests
import json
url= "https://{{instance}}.quable.com.quable.com/api/classifications"
payload=json.dumps({
"id": "classification_sweaters",
"parent": {
"id": "classification_tops"
},
"active": True,
"attributes": {
"quable_classification_name": {
"en_US": "Sweaters",
"fr_FR": "Pulls"
}
}
})
headers= {
'Content-Type': 'application/json',
'Authorization': 'Bearer ...'
}
response= requests.request("POST", url, headers=headers, data=payload)
#
Edit Classifications
To edit a classification, you must know the classification's unique identifier.
#
Endpoint
PUT /api/classifications/[identifier]
This endpoint is unitary
This endpoint is unitary and modifies a single resource at a time
#
Query parameters
#
Body parameters
#
Example
import requests
import json
url = "https://{{instance}}.quable.com/api/classifications/classification_sweaters"
payload = json.dumps({
"id": "classification_sweaters",
"active": True,
"attributes": {
"quable_classification_name": {
"en_US": "Jumpers",
"fr_FR": "Pullover"
}
}
})
headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer ...'
}
response = requests.request("PUT", url, headers=headers, data=payload)
#
Delete Classifications
It may become necessary at some point to delete a classifcation from Quable PIM (e.g., to synchronize with a change in your ERP).
To delete a classification, you must identify the classification's unique identifier.
What happens when a classification is deleted
- All of its child classifications are also deleted.
- All related objects (documents, variants, assets) become orphans (unclassified).
#
Endpoint
/DELETE
/api/classifications/[identifier]
'identifier' being the unique code of the classification to delete.
#
Example
import requests
url = "https://{{instance}}.quable.com/api/classifications/classification_sweaters"
headers= {
'Content-Type': 'application/json',
'Authorization': 'Bearer ...'
}
response= requests.request("DELETE", url, headers=headers)