#
Import using CSV/XLS files
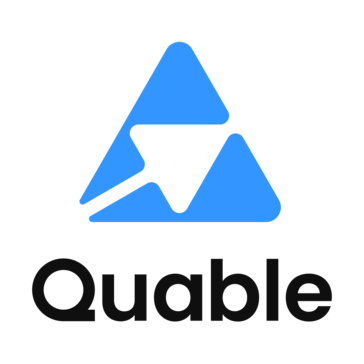
--
--
#
File import order
As for the full import via the API, you are required to import your files following a certain order into Quable PIM in order for the import to be successful.
graph LR A[Push prerequisite values] A --> B[Push classifications] B --> C[Push documents] C --> D[Push variants] D --> E[Push links]
graph LR A[Push prerequisite values] A --> B[Push classifications] B --> C[Push assets] C --> D[Push links]
Prepare you CSV or XLS files in advance before proceeding to their successive import using one of the method described below.
#
Import with file access via https or sftp
#
Scheduled import (through Quable's interface)
You can import your entire product (or media) data into the PIM/DAM in file format (CSV/XLSX) directly from the PIM.
To do so, you will need to (1) create import profiles and then (2) schedule the recurring import of this profile.
Visit our user documentation to learn how to do so from the Quable interface.
This method does not require you to manage any code or orchestration since the PIM retrieves your files at the right time, integrates your data and generates a report. In addition, you can find all imported files and reports in the activity reports.
However, there are a few constraints with this method:
- in order to retrieve your file, our import engine must have access to the file via an HTTP(s) or (s)FTP link.
- You must therefore be able to offer these accesses within the import profile.
- HTTP(s) links must be public.
We do not give a specific IP n order to allow access to our private network. - The (s)FTP server must be freely accessible via a domain/ip, a port, a user and a password.
#
On demand API-driven import
If you are interested in importing files via https
or sftp
, but do not want to use the Quable PIM schedule, it is possible to trigger the import on demand using the API. To do this, you need to create a profile for your import and then use the Quable API to trigger the import.
Here is an example in python:
import requests
import json
url = "https://{{instance}}.quable.com/api/imports"
payload = json.dumps({
"importProfileId": "xxx-xxx-xxxx-xxxx-xxx-xxx",
"remotePath": "sftp://_DOMAIN_.com:__PORT_/_PATH_/_FILENAME_"
})
headers = {
'Content-Type': 'application/hal+json',
'Authorization': 'Bearer _MYAPITOKEN_'
}
response = requests.request("POST", url, headers=headers, data=payload)
In case you want to request the import via an https (instead of sftp) file, you just have to modify the remotePath
value.
You can use the Full Access Token available on your instance.
#
API triggered import with file information on request
If you don't want, or can't use https
or sftp
access to your file, you can create an import profile and launch the import on demand while pushing your file. The latter should be available locally.
To do so, you will need to:
- Push your file to the PIM via the
/api/files
API - Use the
id
returned in the response to launch the import.
Here is an example in python:
import requests
import json
urlFile = "https://{{instance}}.quable.com/api/files"
urlImport = "https://{{instance}}.quable.com/api/imports"
headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer _MYAPITOKEN_'
}
# Push the local file
payload = {'fileType': 'import'}
files=[
(
'file',
('__FILENAME___',open('__PATH_TO_LOCAL_FILE__','rb'),'text/csv')
)
]
responseFile = requests.request("POST", urlFile, headers=headers, data=payload, files=files, timeout=30)
# Retrieve the file ID that will be used when starting the import
fileId = responseFile['id']
# equivalent with CURL
# curl --location urlFile \
# --header 'Authorization: Bearer _MYAPITOKEN_' \
# --form 'file=@"__PATH_TO_LOCAL_FILE__"' \
# --form 'fileType="import"'
# Start the import
payloadImport = json.dumps({
"importProfileId": "__IMPORT-PROFILE-ID__",
"fileId": fileId
})
responseImport = requests.request("POST", urlImport, headers=headers, data=payloadImport, timeout=30)
You can use the Full Access Token available on your instance.