#
Delete all documents, variants & assets
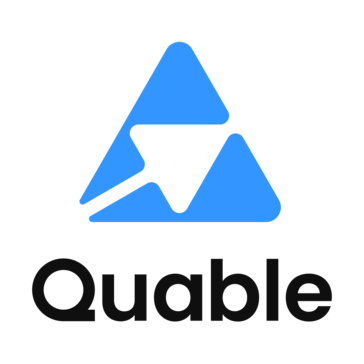
-
-
--
--
--
#
How can I delete all the documents, variants and assets ?
You might need to empty your PIM from all content. There are two ways to delete documents, assets and variants: from Quable's interface (one by one only for now), or through the API.
#
From Quable PIM's interface
You will have to go through each object page and click on "delete" button.
There are currently no other way to delete items in the PIM.
Bulk delete feature
A Bulk-Delete feature is on the Quable roadmap and will soon be available.
#
Through the API
The API allows:
- to identify all items with a GET/api/documents request
- and/or to remove items one by one using their ID with DELETE /api/documents/{{code}} requests
#
Delete all documents
Code snippet file
You can access a Python example on Github here
# Importing necessary libraries
import argparse # Library for parsing command-line arguments
import requests # Library for making HTTP requests
import json # Library for working with JSON data
# Parsing command-line arguments
parser = argparse.ArgumentParser(description='Remove all documents from a given instance')
parser.add_argument('--instance', type=str, help='SubDomain of your QuablePIM', required=True)
parser.add_argument('--token', type=str, help='Access Token with full permissions', required=True)
args = parser.parse_args()
# Initializing variables
documentCodes = [] # List to store document IDs
urlGet = "https://{}.quable.com/api/documents?limit=25" # URL for retrieving documents
urlDelete = "https://{}.quable.com/api_1.php/documents/{}" # URL for deleting documents
headers = {
'Authorization': 'Bearer {}'.format(args.token) # Authorization header with access token
}
# Retrieving all documents
print("Retrieve ALL documents - {}".format(urlGet.format(args.instance)))
response = requests.request("GET", urlGet.format(args.instance), headers=headers)
response_dict = json.loads(response.text)
# Loop to retrieve documents in chunks
for document in response_dict["hydra:member"]:
documentCodes.append(document["id"])
while "hydra:view" in response_dict and "hydra:next" in response_dict["hydra:view"]:
urlNext = "https://{}.quable.com{}".format(args.instance, response_dict["hydra:view"]["hydra:next"])
print(" - Retrieve ALL documents - {}".format(urlNext))
response = requests.request("GET", urlNext, headers=headers)
response_dict = json.loads(response.text)
for document in response_dict["hydra:member"]:
documentCodes.append(document["id"])
# Deleting documents one by one
print("Delete documents one by one")
for documentCode in documentCodes:
print(" - Delete item : '{}'".format(documentCode))
response = requests.request("DELETE", urlDelete.format(args.instance, documentCode), headers=headers)
# Script completion
print('END')
To execute this script, use:
$ python3 QUABLE-PIM_documents-delete-all.py --instance=MINE --token=MY-TOKEN
#
Delete all assets
Code snippet file
You can access a Python example on Github here
# Importing necessary libraries
import argparse # Library for parsing command-line arguments
import requests # Library for making HTTP requests
import json # Library for working with JSON data
# Parsing command-line arguments
parser = argparse.ArgumentParser(description='Remove all assets from a given instance')
parser.add_argument('--instance', type=str, help='SubDomain of your QuablePIM', required=True)
parser.add_argument('--token', type=str, help='Access Token with full permissions', required=True)
args = parser.parse_args()
# Initializing variables
assetCodes = [] # List to store asset IDs
urlGet = "https://{}.quable.com/api/assets?limit=25" # URL for retrieving assets
urlDelete = "https://{}.quable.com/api_1.php/assets/{}" # URL for deleting assets
headers = {
'Authorization': 'Bearer {}'.format(args.token) # Authorization header with access token
}
# Retrieving all assets
print("Retrieve ALL assets - {}".format(urlGet.format(args.instance)))
response = requests.request("GET", urlGet.format(args.instance), headers=headers)
response_dict = json.loads(response.text)
# Loop to retrieve assets in chunks
for asset in response_dict["hydra:member"]:
assetCodes.append(asset["id"])
while "hydra:view" in response_dict and "hydra:next" in response_dict["hydra:view"]:
urlNext = "https://{}.quable.com{}".format(args.instance, response_dict["hydra:view"]["hydra:next"])
print(" - Retrieve ALL assets - {}".format(urlNext))
response = requests.request("GET", urlNext, headers=headers)
response_dict = json.loads(response.text)
for asset in response_dict["hydra:member"]:
assetCodes.append(asset["id"])
# Deleting assets one by one
print("Delete assets one by one")
for assetCode in assetCodes:
print(" - Delete item : '{}'".format(assetCode))
response = requests.request("DELETE", urlDelete.format(args.instance, assetCode), headers=headers)
# Script completion
print('END')
To execute this script, use:
$ python3 QUABLE-PIM_assets-delete-all.py --instance=MINE --token=MY-TOKEN
#
Delete all variants
Code snippet file
You can access a Python example on Github here
To execute this script, use:
$ python3 delete-all-variants.py --instance=MINE --token=MY-TOKEN